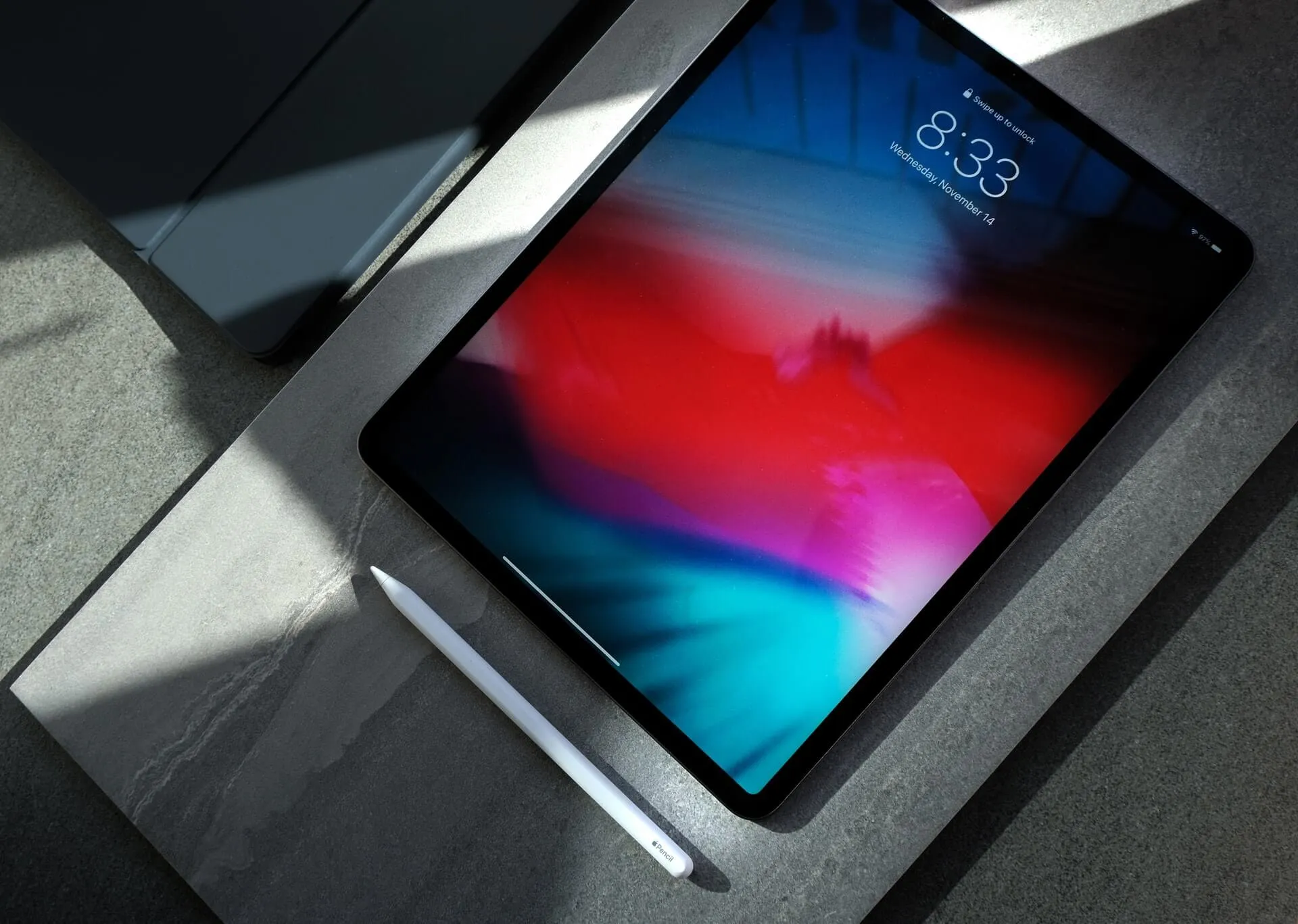
React Native - Create custom iPad screen
Last week I was improving my own React Native application. It works great on mobile devices. However, I wanted to bring support for iPad because all the screens could allow fewer steps. And we can take advantage of side menus of it.
But in the current mobile flow, I have different screens for executing a specific task. The goal of a custom iPad screen is to put a list (HTTP requests in this case) to the left and the content screen to the right.
Since I’m using the React Native Navigation. The only solution that I found is to create a custom screen only for iPad devices. But can React Native detect if a device is an iPad? Well, here is the solution.
The isPad value
This value is only available if Platform
is an iOS device. So be careful with it and add a validation method before.
Solution
import { Platform } from "react-native";import IosMobileScreen from "your-mobile-screen";import IosTabletScreen from "your-tablet-screen";import AndroidScreen from " your-android-screen";
const MainScreen = () => { const isIos = Platform.OS === "ios"; let isTablet = false;
if (isIos) { isTablet = Platform.isPad; }
return isIos ? isTablet ? <IosTabletScreen /> : <IosMobileScreen /> : <AndroidScreen />;};
export default MainScreen;
I tried to make the code longer for better understanding. And assuming that you have three different screen components. One for mobile iOS devices, another for iPad and the last if you want a custom Android screen.
So, first, we validate if the device is really an iOS device, and we prepare the isTablet
variable as false
default. We only enter the conditional if it matches the iOS condition. The Platform.isPad
constant is a boolean. Once we have that value as true
, we can render our iPad component. Only for the example, I named it IosTabletScreen
. We have the other two components if no condition was validated and returns whether it is an Android device or a mobile iOS device.