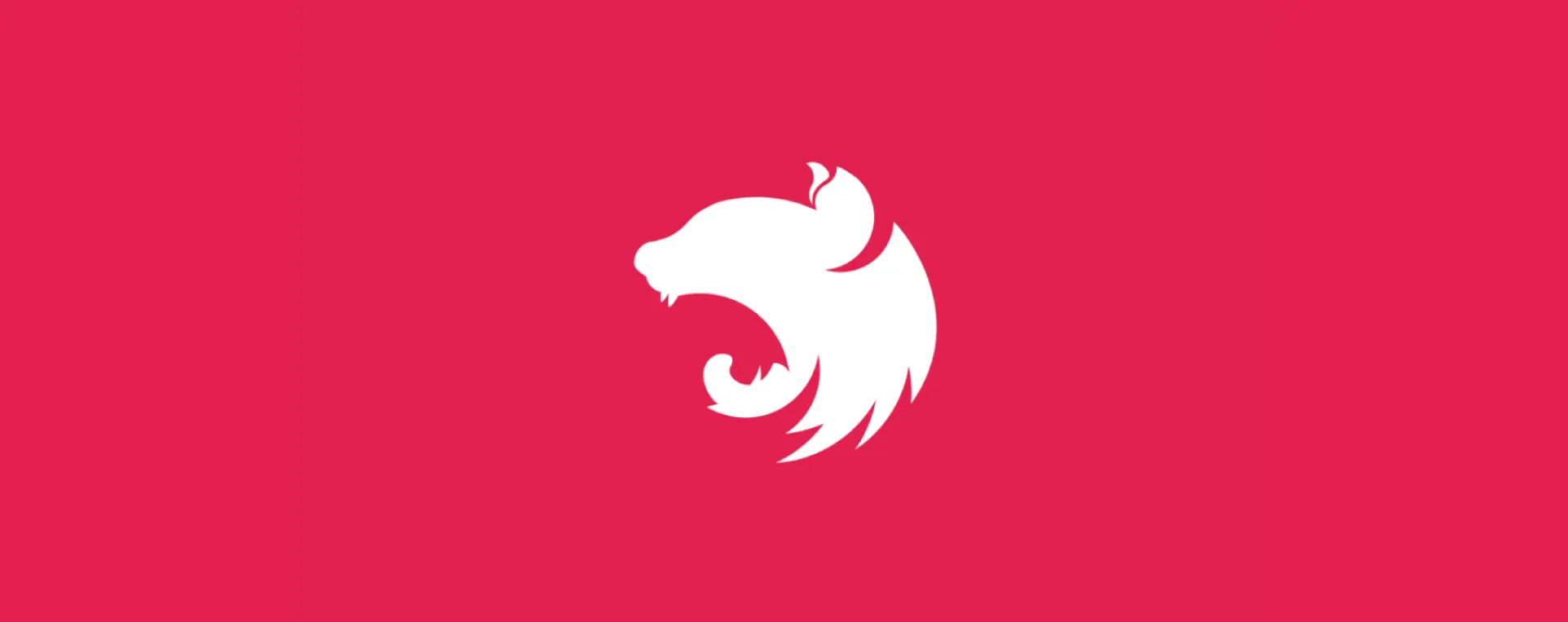
Why Nest JS is one of the best Node backend frameworks?
The JavaScript community is one of the most significant development communities globally. It is not surprising that it is one of the most used languages every year.
Whether you are developing for Backend, you have many options to choose from. If you want to code for Backend, the most popular choice that many developers go for is ExpressJS. You install a couple of libraries in your package.json
, and now you can start creating your API or views that return HTML from the server.
So when reading the title, you may ask yourself: why do I prefer NestJS if there are many other more popular options like Express or Hapi?
Here, I will give you some reasons why I have worked on some projects in NestJS and would use it again if it is a requirement to use NestJS again.
It’s NodeJS (Javascript)
We all know the advantages that Node.js offers as a framework in the backend: speed of execution and the learning curve for people who come from the frontend.
Node.js has become one of the most popular technologies to develop backend applications in recent years. This is because frontend developers feel more comfortable working on the backend with a coding language that they already know.
# Installing NestJS globallynpm i -g @nestjs/cli# Creating a new projectnest new project-name
It recommends, but doesn’t force a specified order for work
And it reminds me of Laravel (PHP).
Let me explain it. The folder organization looks a lot like Angular. It doesn’t force you to sort your code as they put it in the tutorials, but I’ve found that a particular way of organizing the files is a combination of MVC and the Angular framework. Anyone coming from a backend framework other than ExpressJS will feel more comfortable transitioning to Nest.
// A regular controller file@Controller("users")export class UsersController { constructor(private readonly usersService: UsersService) {}
@Post() create(@Body() createUserDto: CreateUserDto) { return this.usersService.create(createUserDto); }
@Get() findAll() { return this.usersService.findAll(); }
@Get(":id") findOne(@Param("id") id: string) { return this.usersService.findOne(+id); }
@Patch(":id") update(@Param("id") id: string, @Body() updateUserDto: UpdateUserDto) { return this.usersService.update(+id, updateUserDto); }
@Delete(":id") remove(@Param("id") id: string) { return this.usersService.remove(+id); }}
Databases and ORMs
Nest works pretty well with almost any popular database engine. Whether you are using MySQL, PostgreSQL, MongoDB, etc.
It also comes with support for different ORMs like TypeORM, Sequelize, MikroORM, Prisma, etc.
The tables or schemas from the database can be created with TypeScript, which is pretty useful to avoid syntax errors.
// A DB model using TypeORM defined with the power of Typescriptimport { Entity, Column, PrimaryGeneratedColumn } from "typeorm";
@Entity()export class Photo { @PrimaryGeneratedColumn() id: number;
@Column({ length: 500 }) name: string;
@Column("text") description: string;
@Column() filename: string;
@Column("int") views: number;
@Column() isPublished: boolean;}
Typescript support
Since 2020, developers who use TypeScript have been on the rise, and I think it is due to the size of current projects and the “state” of the frontend. There is a lot of data to connect between both sides of the development: back and front. It’s necessary to establish specific standards so team members won’t be confused about what type of variables or functions should be used throughout the project. It is there that TypeScript can start to shine with its autocomplete support.
When NestJS uses TypeScript, the ease of writing code is greatly increased, and so are errors in data types before compilation.
Documentation
What I love most about a framework is the documentation and examples that are presented on its website. Confusing documentation is one of the main reasons why a junior to mid-level developer may not feel motivated to work with a web framework, so they tend to avoid using it. I believe NestJS fulfills this requirement. It has one of the best backend framework documentations I have seen, along with other projects like Laravel.
Fast development environments with SWC
NestJS comes with support for SWC, a compilation tool made in Rust, that can increase the speed of compilation by 20 times faster than the TypeScript default compiler.
Support for microservices or serverless applications
NestJS is not meant to be deployed only to any VPS server. It also supports deployment in containers and takes advantage of serverless architecture.
It even supports the RPC protocol, which is useful for communicating between microservices. It uses the gRPC library.
Also, if you want to deploy your application on a serverless provider like AWS Lambda and avoid loading all the NestJS libraries, NestJS allows you to do it.
// How to set up an application in RPC modeconst app = await NestFactory.createMicroservice<MicroserviceOptions>(AppModule, { transport: Transport.GRPC, options: { package: "hero", protoPath: join(__dirname, "hero/hero.proto"), },});
Websockets
If you need real-time connections for your application, NestJS comes with WebSocket support. It supports asynchronous responses, multiple responses, etc.
OpenAPI support with Swagger
If you need to document your API, NestJS can connect to Swagger. Swagger also lets you try the endpoints directly from the browser without any API testing tool like Postman.
It’s also useful if you want to share endpoints with other teams.
// Adding Swagger to your appimport { NestFactory } from "@nestjs/core";import { SwaggerModule, DocumentBuilder } from "@nestjs/swagger";import { AppModule } from "./app.module";
async function bootstrap() { const app = await NestFactory.create(AppModule);
const config = new DocumentBuilder() .setTitle("Cats example") .setDescription("The cats API description") .setVersion("1.0") .addTag("cats") .build(); const document = SwaggerModule.createDocument(app, config); SwaggerModule.setup("api", app, document);
await app.listen(3000);}bootstrap();
GraphQL support
If you need to integrate GraphQL, NestJS provides some easy steps to do so. Just remember that in this approach, you will need to move away from REST controllers and instead use GraphQL resolvers.
import { Module } from "@nestjs/common";import { GraphQLModule } from "@nestjs/graphql";import { ApolloDriver, ApolloDriverConfig } from "@nestjs/apollo";
@Module({ imports: [ GraphQLModule.forRoot<ApolloDriverConfig>({ driver: ApolloDriver, }), ],})export class AppModule {}
Devtools
NestJS recently added a developer tool that allows you to check the application’s organization in real time. You can see how your modules and services are connected to each other and avoid circular dependencies.
@Module({ imports: [ DevtoolsModule.register({ http: process.env.NODE_ENV !== "production", }), ], controllers: [AppController], providers: [AppService],})export class AppModule {}
Task scheduler (Crons)
When you need to run tasks in the background, you don’t need a cron job running on your server. NestJS allows you to do it with a decorator.
This feature is also useful if you need to execute automatic tasks, such as sending an email every few specific hours or updating your database information at any time of the day.
import { Injectable, Logger } from "@nestjs/common";import { Cron } from "@nestjs/schedule";
@Injectable()export class TasksService { private readonly logger = new Logger(TasksService.name);
@Cron("45 * * * * *") handleCron() { this.logger.debug("Called when the current second is 45"); }}
Caching
If you don’t want to query the database for pieces of information that are not updated frequently, NestJS adds cache support to your application.
It doesn’t only add cache support to REST endpoints, but also to Websockets and Microservices.
@Controller()export class AppController { @CacheKey("custom_key") @CacheTTL(20) findAll(): string[] { return []; }}
Encrypting and hashing
When you need to exchange data between different microservices or application parts, it is important to consider the security of the information, as it can be intercepted. In NestJS, you can encrypt data to ensure its confidentiality.
NestJS utilizes the native cryptography library from NodeJS, called crypto
.
// Example of encrypting dataimport { createCipheriv, randomBytes, scrypt } from "crypto";import { promisify } from "util";
const iv = randomBytes(16);const password = "Password used to generate key";
const key = (await promisify(scrypt)(password, "salt", 32)) as Buffer;const cipher = createCipheriv("aes-256-ctr", key, iv);
const textToEncrypt = "Nest";const encryptedText = Buffer.concat([cipher.update(textToEncrypt), cipher.final()]);
And more features
NestJS offers several other features that have not been mentioned in this article. Here is a quick list of these features that you may find useful:
- Sessions
- CSRF protection
- Pipes
- Creation of CLI applications
- Healthchecks
- Support for NATS, MQTT, Kafka, RabbitMQ, etc.
Conclusion
In my opinion, these are the main reasons to choose NestJS for any new project requiring Node for the backend. Without a doubt, I would recommend using NestJS. It provides additional features that make backend development as simple and fun as possible. I encourage you to explore these features further in the documentation .